The C++ Interview is highly regarded among tech enthusiasts and is a dream job for many. With the increasing number of software jobs available, more people are choosing this career path, leading to stiff competition. Landing a C++ job isn’t as simple as it seems; you need strong qualifications, good grades, and relevant courses. Then comes the nerve-wracking interview, where the employer assesses if you’re the right fit for the job.
While candidates can handle most aspects of job hunting, the interview tends to be the most intimidating. There’s a lot that can go wrong, making it the scariest part of the process. That’s why many people seek assistance to better prepare themselves for the upcoming interview.
In this article, we’ve compiled a list of commonly asked C++ interview questions. These questions will not only help you prepare for the interview but also boost your confidence. Take a look and get ready for your next C++ interview!
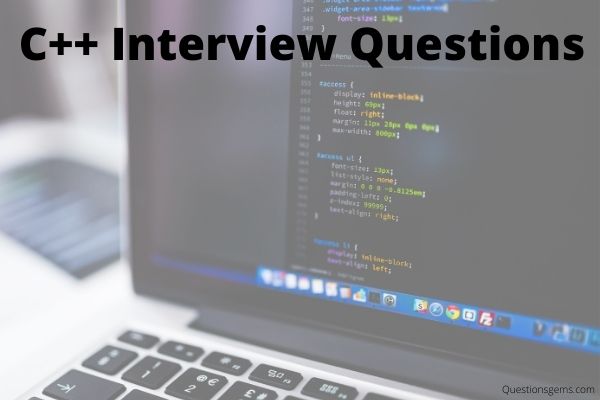
C++ Interview Questions
Q.What is this pointer?Ans.The ‘this’ pointer is passed as a hidden argument to all nonstatic member function calls and is available as a local variable within the body of all nonstatic functions. ‘this’ pointer is a constant pointer that holds the memory address of the current object. ‘this’ pointer is not available in static member functions as static member functions can be called without any object (with class name).
1.C++ is a kind of superset of C, most of C programs except few exceptions (See this and this) work in C++ as well.
2.C is a procedural programming language, but C++ supports both procedural and Object Oriented programming.
3.Since C++ supports object oriented programming, it supports features like function overloading, templates, inheritance, virtual functions, friend functions. These features are absent in C.
4.C++ supports exception handling at language level, in C exception handling is done in traditional if-else style.
5.C++ supports references, C doesn’t.
6.In C, scanf() and printf() are mainly used input/output. C++ mainly uses streams to perform input and output operations. cin is standard input stream and cout is standard output stream.
Q.What are VTABLE and VPTR?Ans.vtable is a table of function pointers. It is maintained per class.vptr is a pointer to vtable. It is maintained per object (See this for an example).Compiler adds additional code at two places to maintain and use vtable and vptr.1) Code in every constructor. This code sets vptr of the object being created. This code sets vptr to point to vtable of the class.2) Code with polymorphic function call (e.g. bp->show() in above code). Wherever a polymorphic call is made, compiler inserts code to first look for vptr using base class pointer or reference (In the above example, since pointed or referred object is of derived type, vptr of derived class is accessed). Once vptr is fetched, vtable of derived class can be accessed. Using vtable, address of derived derived class function show() is accessed and called.
Q. Major Differences between JAVA and C++Ans.There are lot of differences, some of the major differences are:–Java has automatic garbage collection whereas C++ has destructors , which are automatically invoked when the object is destroyed.–Java does not support pointers, templates, unions, operator overloading, structures etc.C++ has no in built support for threads,whereas in Java there is a Thread class that you inherit to create a new threadNo goto in JAVA–C++ support multiple inheritance, method overloading and operator overloading but JAVA only has method overloading.–Java is interpreted and hence platform independent whereas C++ isn’t. At compilation time, Java Source code converts into JVM byte code. The interpreter execute this bytecode at run time and gives output. C++ run and compile using compiler which converts source code into machine level language.
Q.What are C++ access specifiers ?Ans.Access specifiers are used to define how the members (functions and variables) can be accessed outside the class.–Private: Members declared as private are accessible only within the same class and they cannot be accessed outside the class they are declared. Child classes are also not allowed to access private members of parent.Public: Members declared as public are accessible from anywhere.Protected: Only the class and its child classes can access protected members.
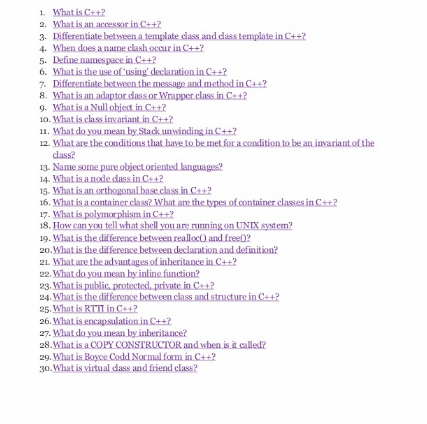
Q.What Is Inheritance?Ans.Different kinds of objects often have a certain amount in common with each other. Yet each also defines additional features that make them different. Object-oriented programming allows classes to inherit commonly used state and behavior from other classes
Q. What is Static Member?Ans.Static is a keyword in C++ used to give special characteristics to an element. Static elements are allocated storage only once in a program lifetime in static storage area. And they have a scope till the program lifetime.
C++ Interview Questions For Experienced
Question. What Is The Difference Between Exit And Abort?Answer :exit does a graceful process termination, it calls the destructors for all the constructed objects, with abort they are not called.With exit the local With variables of the calling function and its callers will not have their destructors invoked.
Question. Can I Have Static Members In An Union?Answer :No
Question. Can I Overload Destructor?Answer :No
Question. Can I Call Destructor Explicitly?Answer :Yes, but you only want to do that when you have used placement new.
Question. Where Virtual Inheritance Should Be Used In A Hierarchy?Answer :If we have a diamond class hierarchy we should use the virtual inheritance just below the top of the diamond.
Question. How Do You Link A C++ Program To C Functions?Answer :By using the extern “C” linkage specification around the C function declarations.
Question. Explain The Scope Resolution Operator?Answer :It permits a program to reference an identifier in the global scope that has been hidden by another identifier with the same name in the local scope.
Question. What Are The Differences Between A C++ Struct And C++ Class?Answer :The default member and base-class access specifiers are different.
Question. How Many Ways Are There To Initialize An Int With A Constant?Answer :Two. There are two formats for initializers in C++ as shown in the example that follows.The first format uses the traditional C notation.The second format uses constructor notation.int foo = 123;int bar (123);
Question. How Does Throwing And Catching Exceptions Differ From Using Setjmp And Longjmp?Answer :The throw operation calls the destructors for automatic objects instantiated since entry to the try block.
Question. What Is Your Reaction To This Line Of Code?Answer :It’s not a good practice.
Question. What Is A Conversion Constructor?Answer :A constructor that accepts one argument of a different type.
Question. What Is The Difference Between A Copy Constructor And An Overloaded Assignment Operator?Answer :A copy constructor constructs a new object by using the content of the argument object. An overloaded assignment operator assigns the contents of an existing object to another existing object of the same class.
Question. When Should You Use Multiple Inheritance?Answer :There are three acceptable answers: “Never,” “Rarely,” and “When the problem domain cannot be accurately modeled any other way.”
Question. What Is A Virtual Destructor?Answer :The simple answer is that a virtual destructor is one that is declared with the virtual attribute.
Question. Explain The Isa And Hasa Class Relationships. How Would You Implement Each In A Class Design?Answer :A specialized class “is” a specialization of another class and, therefore, has the ISA relationship with the other class. An Employee ISA Person. This relationship is best implemented with inheritance. Employee is derived from Person. A class may have an instance of another class. For example, an employee “has” a salary, therefore the Employee class has the HASA relationship with the Salary class. This relationship is best implemented by embedding an object of the Salary class in the Employee class.
Question. When Is A Template A Better Solution Than A Base Class?Answer :When you are designing a generic class to contain or otherwise manage objects of other types, when the format and behavior of those other types are unimportant to their containment or management, and particularly when those other types are unknown (thus, the genericity) to the designer of the container or manager class.
Question. What Is A Mutable Member?Answer :One that can be modified by the class even when the object of the class or the member function doing the modification is const.
Question. What Is An Explicit Constructor?Answer :A conversion constructor declared with the explicit keyword. The compiler does not use an explicit constructor to implement an implied conversion of types. It’s purpose is reserved explicitly for construction.
Question. What Is The Standard Template Library?Answer :A library of container templates approved by the ANSI committee for inclusion in the standard C++ specification.A programmer who then launches into a discussion of the generic programming model, iterators, allocators, algorithms, and such, has a higher than average understanding of the new technology that STL brings to C++ programming.
Question. Describe Run-time Type Identification.?Answer :The ability to determine at run time the type of an object by using the typeid operator or the dynamic_cast operator.
Question. What Problem Does The Namespace Feature Solve?Answer :Multiple providers of libraries might use common global identifiers causing a name collision when an application tries to link with two or more such libraries. The namespace feature surrounds a library’s external declarations with a unique namespace that eliminates the potential for those collisions. This solution assumes that two library vendors don’t use the same namespace identifier, of course.
Question. Are There Any New Intrinsic (built-in) Data Types?Answer :Yes. The ANSI committee added the bool intrinsic type and its true and false value keywords.
C++ Interview Questions And Answers
Question 1. How To Initialize Constant And Reference Member Variable?Answer :Using initialization list.
Question 2. What Is Constant In A Const Function?Answer :Variable ‘this’.
Question 3. What Is The Issue In The Following Program?Answer :#include <iostream>int main(int argc, char **argv){const int & r1 = 100;int v = 200;int &r2 = v;int & r3 = 200;return 0;}Issue is in the initialization of r3 at line 8, rvalue should be a variable.
Question 4. Can The Destructor Be Pure Virtual Function?Answer :Yes, but you still have to define it!
Question 5. What Is The Memory Structure Of An Object?Answer :Usually C++ objects are made by concatenating member variables.For example;class Test{int i;float j;};is represented by an int followed by a float.class TestSub: public Test{int k;};The above class is represented by Test and then an int(for int k). So finally it will be int, float and int.In addition to this each object will have the vptr(virtual pointer) if the class has virtual function, usually as the first element in a class.
Question 6. What Is The Difference Between Std::vector<int> X; And Std::vector<int> X();?Answer :First one declares a variable x of type std::vector<int>. Second one declares a function x which returns std::vector<int>.
Question 7. What Is A Default Constructor?Answer :A constructor which takes no argumentA constructor which has argument(s) but is(are) with default value
Question 8. Can I Use This Pointer In The Constructor?Answer :Yes, but try to avoid calling virtual function from the constructor and passing this pointer from the initialization list to other classes.
Question 9. Does Friends Are Inherited?Answer :No
Question 10. What Is The Difference Between Calling Just Throw And Throw With An Object In A Catch Block?Answer :A copy of the object is created if we throw with an object. With just throw, no copy is created.
Question 11. What Is A Possible Replacement For C Static Function In C++?Answer :Unnamed namespaces.
Question 12. What Is The Size Of An Empty Class, Or Class With Only Normal Functions?Answer :Not zero, 1 for most compilers. The reason for this is to have different address for different object.
Question 13. What Is The Size Of Class With Only Virtual Functions?Answer :4 with most of the compilers for a 32bit binary.
Question 14. How To Declare A Namespace Alias?Answer :namespace MyLongNameSpaceName{…}namespace MLNSN = MyLongNameSpaceName;
Question 15. How To Declare C Function In C++?Answer :By using extern “C”.extern “C” void print();orextern “C” {void print();}
C And C++ Interview Questions And Answers For Experienced
Q. How to free a block of memory previously allocated without using free?Ans:If the pointer holding that memory address is passed to realloc with size argument as zero (like realloc(ptr, 0)) the the memory will be released.
Q. How can you print a string containing ‘%’ in printf?Ans:There are no escape sequence provided for ‘%’ in C. To print ‘%’ one should use ‘%%’, like –printf(“He got 90%% marks in math”);
Q. What is use of %n in printf()?Ans:According to man page “the number of characters written so far is stored into the integer. indicated by the int * (or variant) pointer argument.“. Meaning if we use it in printf, it will get the number of characters already written until %n is encountered and this number will stored in the variable provided. The variable must be an integer pointer.–#include<stdio.h>main(){int c;printf(“Hello%n world “,&c);printf(“%d”, c);}Above program will print ‘Hello world 5 “ as Hello is 5 letter.
Q. What is rvalue and lvalue?Ans:You can think lvalue as a left side operant in an assignment and rvalue is the right. Also, you can remember lavlue as location. So, the lvalue means a location where you can store any value. Say, for statement i = 20, the value 20 is to be stored in the location or address of the variable i. 20 here is rvalue. Then the 20 = I, statement is not valid. It will result in compilation error “lvalue required” as 20 does not represent any location.
Conclusion –
Here are some C++ interview questions you should prepare for. While they may not cover every question you’ll be asked, there’s a good chance you’ll encounter some of these during your interview. These questions have been carefully selected by experts and professionals to give you a strong foundation for your interview preparation. We hope this list of top C++ interview questions helps you get a head start.